UUID Generation in C++11
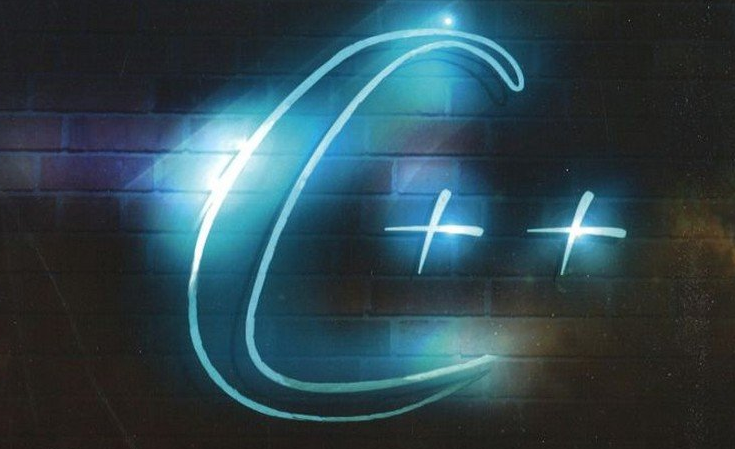
The C++ standard libary has come a long way in the last ten years. It contains a lot of useful functions that make stuff easy that used to be a chore in C++ compared to other languages. However, there is still a gap between what the STL provides compared to other modern languages.
One such case is UUID/GUID creation. In languages like Python and Java, to make a GUID, all you need to do is import a module and you are good to go. In C++, this will still be relegated to an external framework like the Windows API or QT. There are even some smaller cross platform libraries specifically targeting this functionality. In most cases, this is still the best place to go. These methods are battle-tested: they'll be fast and robust.
If you want something more portable that doesn't rely on any external linking, you'll have to write it yourself. Luckily, the UUID format isn't particularly complex. If you can generate random hex characters, you can make a GUID easily.
The STL in C++11 now includes std::random
which makes this a lot easier. Before it, you would still probably need to go to an external library to get random values. In combination with std::hex, almost all of the hard parts of this problem can be solved with stuff in the standard library.
#include <vector>
#include <iostream>
#include <sstream>
#include <random>
#include <climits>
#include <algorithm>
#include <functional>
#include <string>
unsigned char random_char() {
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(0, 255);
return static_cast<unsigned char>(dis(gen));
}
std::string generate_hex(const unsigned int len) {
std::stringstream ss;
for(auto i = 0; i < len; i++) {
auto rc = random_char();
std::stringstream hexstream;
hexstream << std::hex << int(rc);
auto hex = hexstream.str();
ss << (hex.length() < 2 ? '0' + hex : hex);
}
return ss.str();
}
These two functions allow you to generate hex random characters of n
length. From there it is as simple calling it for each of the 5 parts of a UUID by their respective lengths. If you can easily access the UUID functionality in another library, use it. It is likely more performant and can take full advantage of the OS APIs.